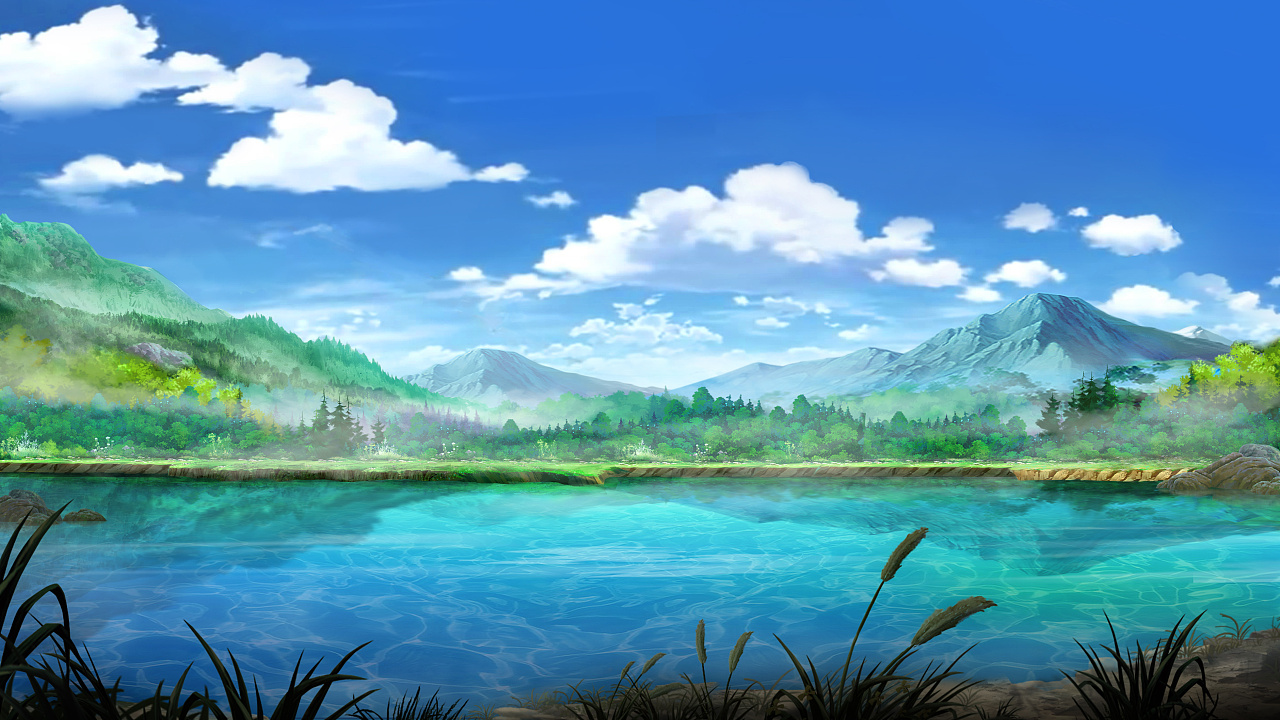
datastruct_5
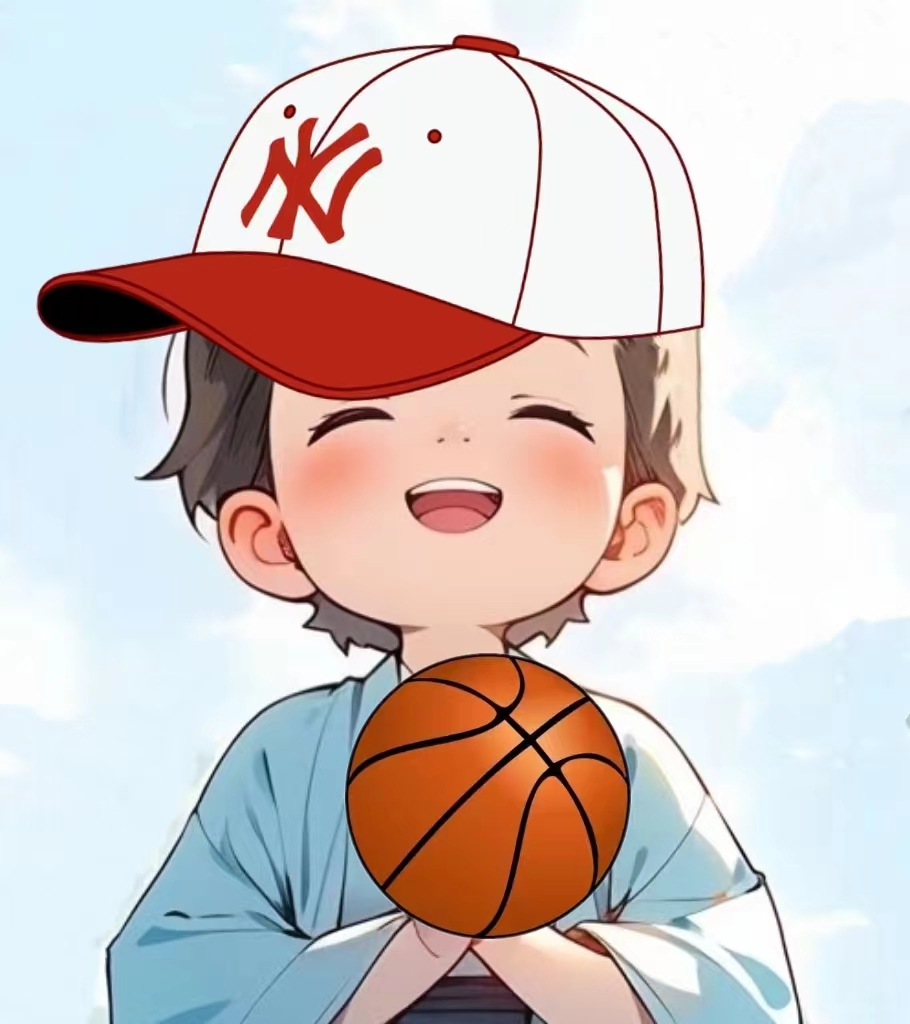
查找相关笔记
1 |
|
- Title: datastruct_5
- Author: Charles
- Created at : 2022-12-29 11:01:12
- Updated at : 2023-07-18 21:14:47
- Link: https://charles2530.github.io/2022/12/29/datastruct-5/
- License: This work is licensed under CC BY-NC-SA 4.0.
recommend_articles
recommend_articles
Comments