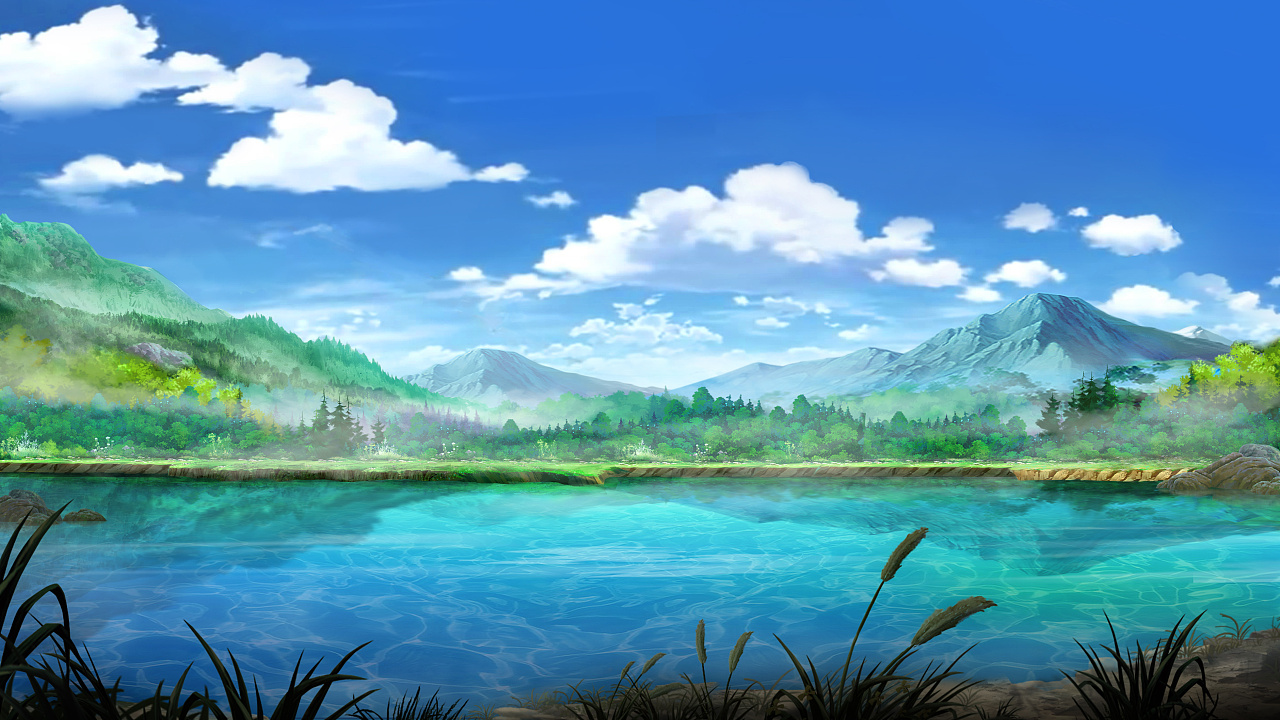
Java_note_1
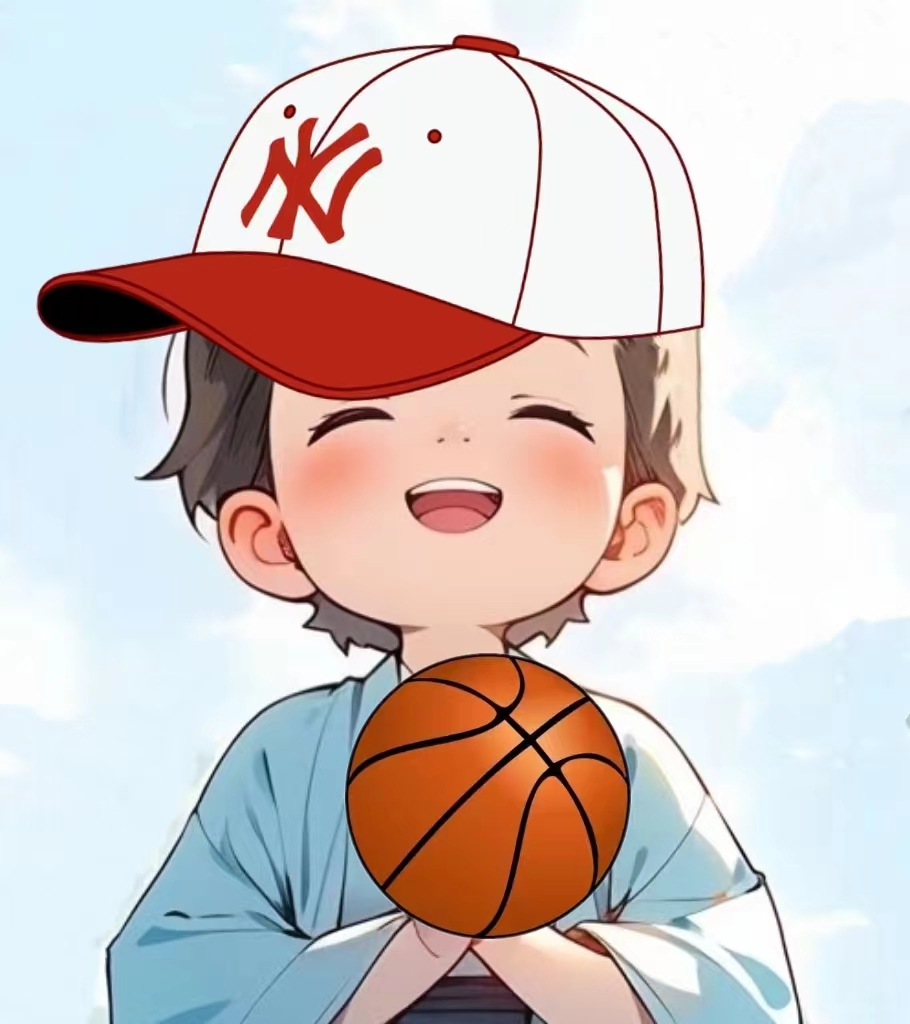
Java_note Basis
1.Collection_Class
ArrayList_Class
1 | package note_file.Basis.Collection_Class; |
Collection_demo1.java
1 | package note_file.Basis.Collection_Class; |
Collections_Class
1 | package note_file.Basis.Collection_Class; |
List_demo1.java
1 | package note_file.Basis.Collection_Class; |
2.Data_Class
Calendar_Class
1 | package note_file.Basis.Date_Class; |
Date_Class
1 | package note_file.Basis.Date_Class; |
SimpleDateFormat_Class
1 | package note_file.Basis.Date_Class; |
3.Map_Class
Map_Class
1 | package note_file.Basis.Map_Class; |
4.Object_Class
Object_Class
1 | package note_file.Basis.Object_Class; |
student.java
1 | package note_file.Basis.Object_Class; |
5.Set_Class
Set_Class
1 | package note_file.Basis.Set_Class; |
HashSet_Class
1 | package note_file.Basis.Set_Class; |
LinkedHashSet_Class
1 | package note_file.Basis.Set_Class; |
Set_demo1.java
1 | package note_file.Basis.Set_Class; |
Student_hash.java
1 | package note_file.Basis.Set_Class; |
TreeSet_Class
1 | package note_file.Basis.Set_Class; |
6.Thread_Class
BOX
BOX
1 | package note_file.Basis.Thread_Class.Box; |
Box.java
1 | package note_file.Basis.Thread_Class.Box; |
Customer.java
1 | package note_file.Basis.Thread_Class.Box; |
Producer.java
1 | package note_file.Basis.Thread_Class.Box; |
MyRunnable_demo.java
1 | package note_file.Basis.Thread_Class; |
MyRunnable.java
1 | package note_file.Basis.Thread_Class; |
MyThread.java
1 | package note_file.Basis.Thread_Class; |
Sell_ticket_demo.java
1 | package note_file.Basis.Thread_Class; |
Sell_ticket.java
1 | package note_file.Basis.Thread_Class; |
Thread_demo1.java
1 | package note_file.Basis.Thread_Class; |
Thread_demo2.java
1 | package note_file.Basis.Thread_Class; |
Thread_Sleep_demo.java
1 | package note_file.Basis.Thread_Class; |
Thread_Sleep.java
1 | package note_file.Basis.Thread_Class; |
7.Other
Array_demo.java
1 | package note_file.Basis.other; |
Arrays_Class
1 | package note_file.Basis.other; |
Constant_value
1 | package note_file.Basis.other; |
Control_Statement
1 | package note_file.Basis.other; |
Input
1 | package note_file.Basis.other; |
Integer_Class
1 | package note_file.Basis.other; |
Math_Class
1 | package note_file.Basis.other; |
Method
1 | package note_file.Basis.other; |
String_Class
1 | package note_file.Basis.other; |
StringBuilder_Class
1 | package note_file.Basis.other; |
System_Class
1 | package note_file.Basis.other; |
- Title: Java_note_1
- Author: Charles
- Created at : 2022-12-29 11:32:01
- Updated at : 2023-02-09 09:45:33
- Link: https://charles2530.github.io/2022/12/29/java-note-1/
- License: This work is licensed under CC BY-NC-SA 4.0.
recommend_articles
recommend_articles
Comments