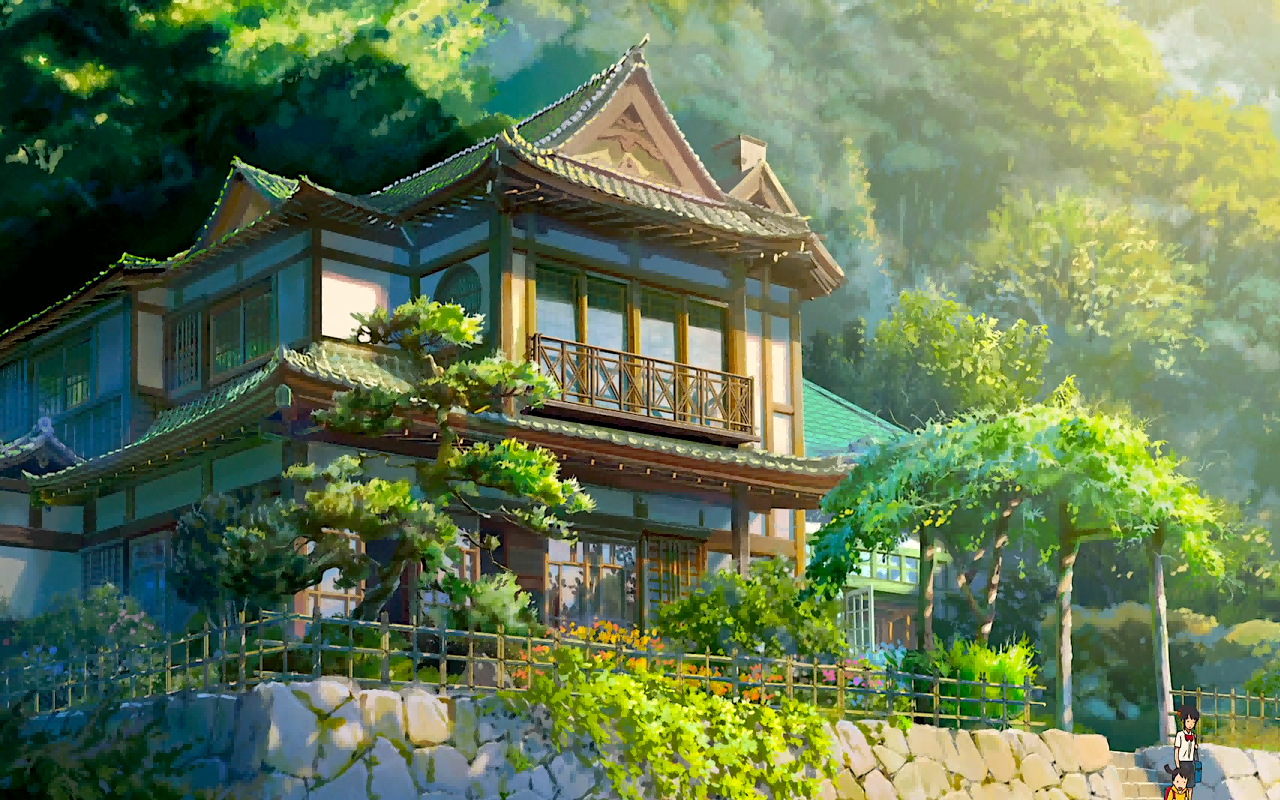
Node-note-1
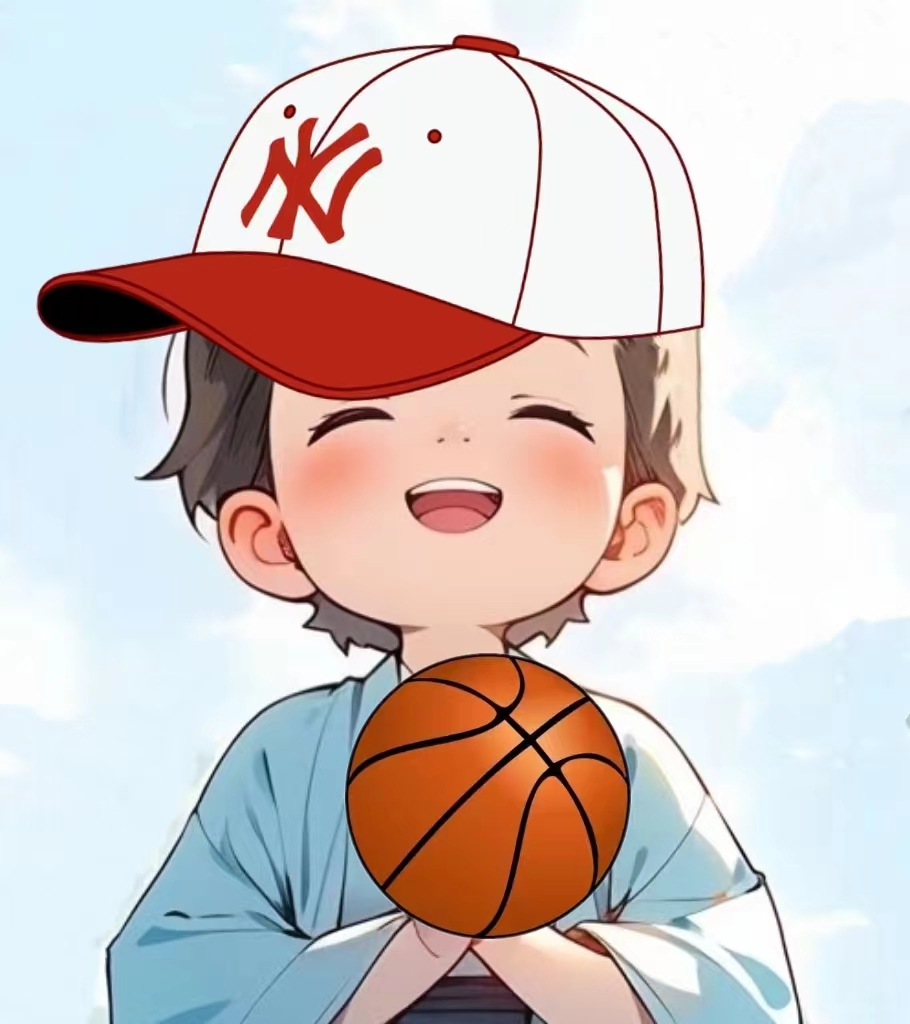
初识Node.js与内置模块
初识 Node.js
Node.js 是一个基于 Chrome V8 引擎的 JavaScript 运行环境。
Node.js 作为一个 JavaScript 的运行环境,仅仅提供了基础的功能和 API。然而,基于 Node.js 提供的这些基础能,很多强大的工具和框架如雨后春笋,层出不穷:
- 基于 Express 框架(http://www.expressjs.com.cn/),可以快速构建 Web 应用
- 基于 Electron 框架(https://electronjs.org/),可以构建跨平台的桌面应用
- 基于 restify 框架(http://restify.com/),可以快速构建 API 接口项目
- 读写和操作数据库、创建实用的命令行工具辅助前端开发
fs 文件系统模块
fs 模块是 Node.js 官方提供的、用来操作文件的模块。它提供了一系列的方法和属性,用来满足用户对文件的操作需求。
例如:
fs.readFile() 方法,用来读取指定文件中的内容
fs.writeFile() 方法,用来向指定的文件中写入内容
如果要在 JavaScript 代码中,使用 fs 模块来操作文件,则需要使用如下的方式先导入它。
fs.readFile()
使用 fs.readFile() 方法,可以读取指定文件中的内容。
1 | // 1. 导入 fs 模块,来操作文件 |
参数解读:
参数1:必选参数,字符串,表示文件的路径。
参数2:可选参数,表示以什么编码格式来读取文件。
参数3:必选参数,文件读取完成后,通过回调函数拿到读取的结果。
fs.writeFile()
使用 fs.writeFile() 方法,可以向指定的文件中写入内容。
1 | // 1. 导入 fs 文件系统模块 |
参数解读:
参数1:必选参数,需要指定一个文件路径的字符串,表示文件的存放路径。
参数2:必选参数,表示要写入的内容。
参数3:可选参数,表示以什么格式写入文件内容,默认值是 utf8。
参数4:必选参数,文件写入完成后的回调函数。
注意:
- fs.writeFile() 方法只能用来创建文件,不能用来创建路径
- 重复调用 fs.writeFile() 写入同一个文件,新写入的内容会覆盖之前的旧内容
路径动态拼接的问题
在使用 fs 模块操作文件时,如果提供的操作路径是以 ./ 或 …/ 开头的相对路径时,很容易出现路径动态拼接错误的问题。
原因:代码在运行的时候,会以执行 node 命令时所处的目录,动态拼接出被操作文件的完整路径。
解决方案:在使用 fs 模块操作文件时,直接提供完整的路径,不要提供 ./ 或 …/ 开头的相对路径,从而防止路径动态拼接的问题。
1 | const fs = require('fs') |
path 路径模块
path 模块是 Node.js 官方提供的、用来处理路径的模块。它提供了一系列的方法和属性,用来满足用户对路径的处理需求。
例如:
path.join() 方法,用来将多个路径片段拼接成一个完整的路径字符串
path.basename() 方法,用来从路径字符串中,将文件名解析出来
如果要在 JavaScript 代码中,使用 path 模块来处理路径,则需要使用如下的方式先导入它。
path.join()
使用 path.join() 方法,可以把多个路径片段拼接为完整的路径字符串。
1 | const path = require('path') |
参数解读:
…paths <string> 路径片段的序列
返回值: <string>
凡是涉及到路径拼接的操作,都要使用 path.join() 方法进行处理。不要直接使用 + 进行字符串的拼接。
path.basename()
使用 path.basename() 方法,可以获取路径中的最后一部分,经常通过这个方法获取路径中的文件名。
1 | const path = require('path') |
参数解读:
path <string> 必选参数,表示一个路径的字符串
ext <string> 可选参数,表示文件扩展名
返回: <string> 表示路径中的最后一部分
path.extname()
使用 path.extname() 方法,可以获取路径中的扩展名部分。
1 | const path = require('path') |
参数解读:
path <string>必选参数,表示一个路径的字符串
返回: <string> 返回得到的扩展名字符串
http 模块
http 模块是 Node.js 官方提供的、用来创建 web 服务器的模块。通过 http 模块提供的 http.createServer() 方法,就能方便的把一台普通的电脑,变成一台 Web 服务器,从而对外提供 Web 资源服务。
如果要希望使用 http 模块创建 Web 服务器,则需要先导入它。
服务器和普通电脑的区别在于,服务器上安装了 web 服务器软件,例如:IIS、Apache 等。通过安装这些服务器软件,就能把一台普通的电脑变成一台 web 服务器。
在 Node.js 中,我们不需要使用 IIS、Apache 等这些第三方 web 服务器软件。因为我们可以基于 Node.js 提供的 http 模块,通过几行简单的代码,就能轻松的手写一个服务器软件,从而对外提供 web 服务。
创建 web 服务器
调用 http.createServer() 方法,即可快速创建一个 web 服务器实例。
1 | // 1. 导入 http 模块 |
为服务器实例绑定 request 事件,即可监听客户端发送过来的网络请求。
调用服务器实例的 .listen() 方法,即可启动当前的 web 服务器实例。
req 请求对象
只要服务器接收到了客户端的请求,就会调用通过 server.on() 为服务器绑定的 request 事件处理函数。如果想在事件处理函数中,访问与客户端相关的数据或属性,可以使用如下的方式:
1 | const http = require('http') |
res 响应对象
在服务器的 request 事件处理函数中,如果想访问与服务器相关的数据或属性,调用 res.end() 方法,向客户端响应一些内容。
1 | const http = require('http') |
- Title: Node-note-1
- Author: Charles
- Created at : 2023-07-24 08:12:31
- Updated at : 2023-07-26 16:22:39
- Link: https://charles2530.github.io/2023/07/24/node-note-1/
- License: This work is licensed under CC BY-NC-SA 4.0.