Cpp_part4
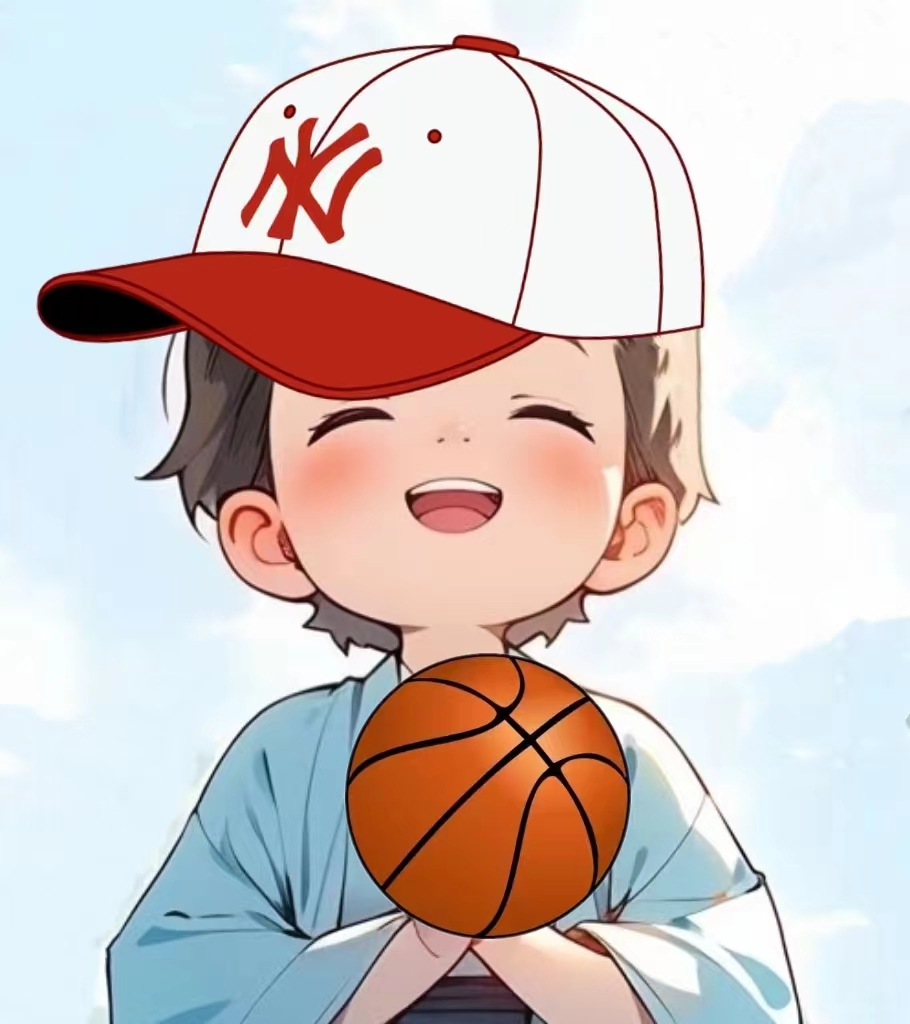
Cpp_part4
1.单键模式
1 | class Single { |
2.reference 引用
1 | class Test { |
3.const
1 | // const与函数返回值的关系 |
Summary
pass by value | pass by address (pointer ,reference) | |
---|---|---|
功能 | read | read/write |
性能 | 低效(sizeof(obj)) | 高效(sizeof(pointer)) |
其他 | copy constructor | nothing |
never pass by value
Main part
1 |
|
- Title: Cpp_part4
- Author: Charles
- Created at : 2022-12-28 08:25:52
- Updated at : 2023-02-09 17:57:11
- Link: https://charles2530.github.io/2022/12/28/cpp-part4/
- License: This work is licensed under CC BY-NC-SA 4.0.
recommend_articles
recommend_articles
Comments