Cpp_part3
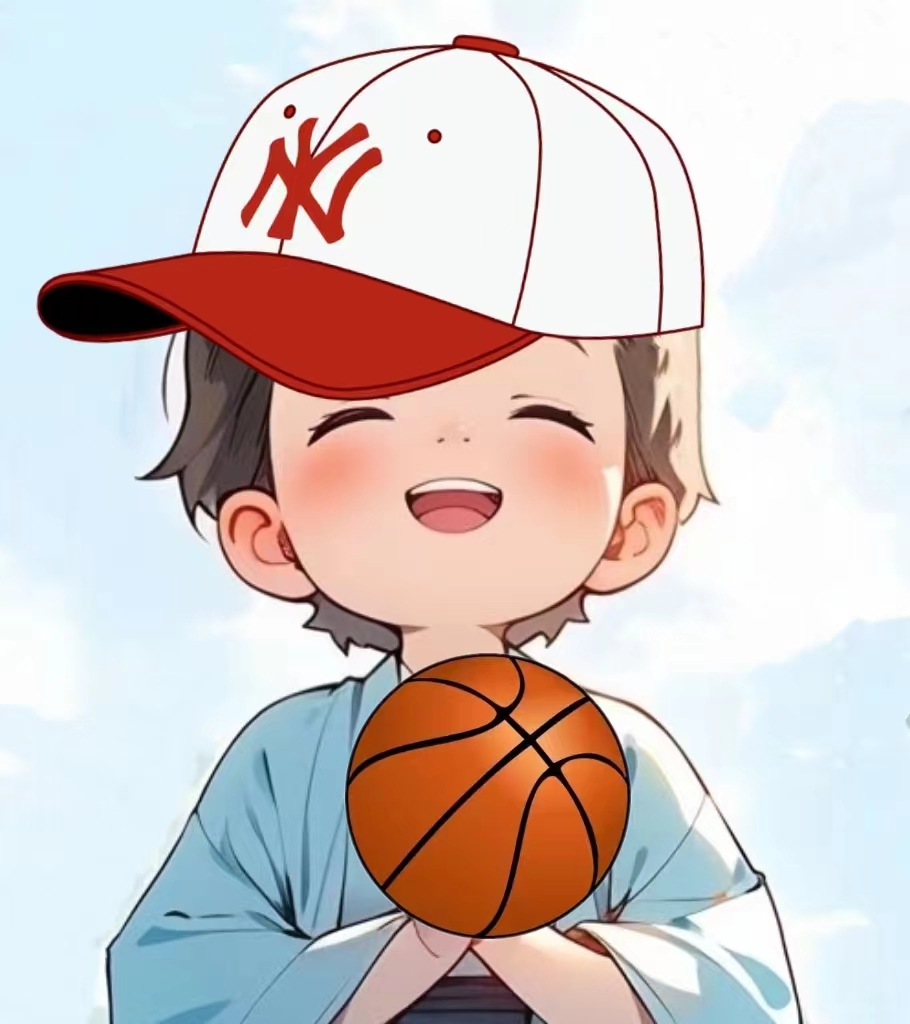
Cpp_part3
1.Constructor and destructor 构造和析构函数
destructor 析构
1 | class Engine {}; |
2.Static 静态变量
static 静态的
Summary
1.static local var 一次构造,最后释放
2.static global function
本文件使用(将函数的可链接范围从默认从全局域限制为文件域)
3.static attribute 本类所有对象的共享内存
4.static method ,可以用本类类名直接调用,但内部不可操作非静态
1 | // static |
3.reference 引用
reference:引用是个安全的指针
1 | void test01() { |
Main part
1 |
|
- Title: Cpp_part3
- Author: Charles
- Created at : 2022-12-28 08:19:33
- Updated at : 2023-02-09 17:57:07
- Link: https://charles2530.github.io/2022/12/28/cpp-part3/
- License: This work is licensed under CC BY-NC-SA 4.0.
recommend_articles
recommend_articles
Comments