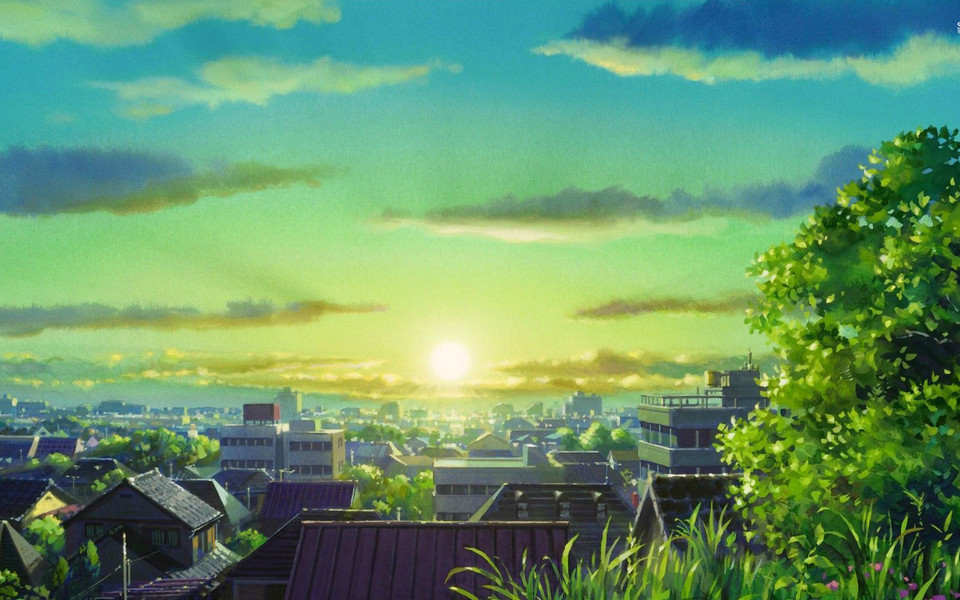
tensorflow-model-summary
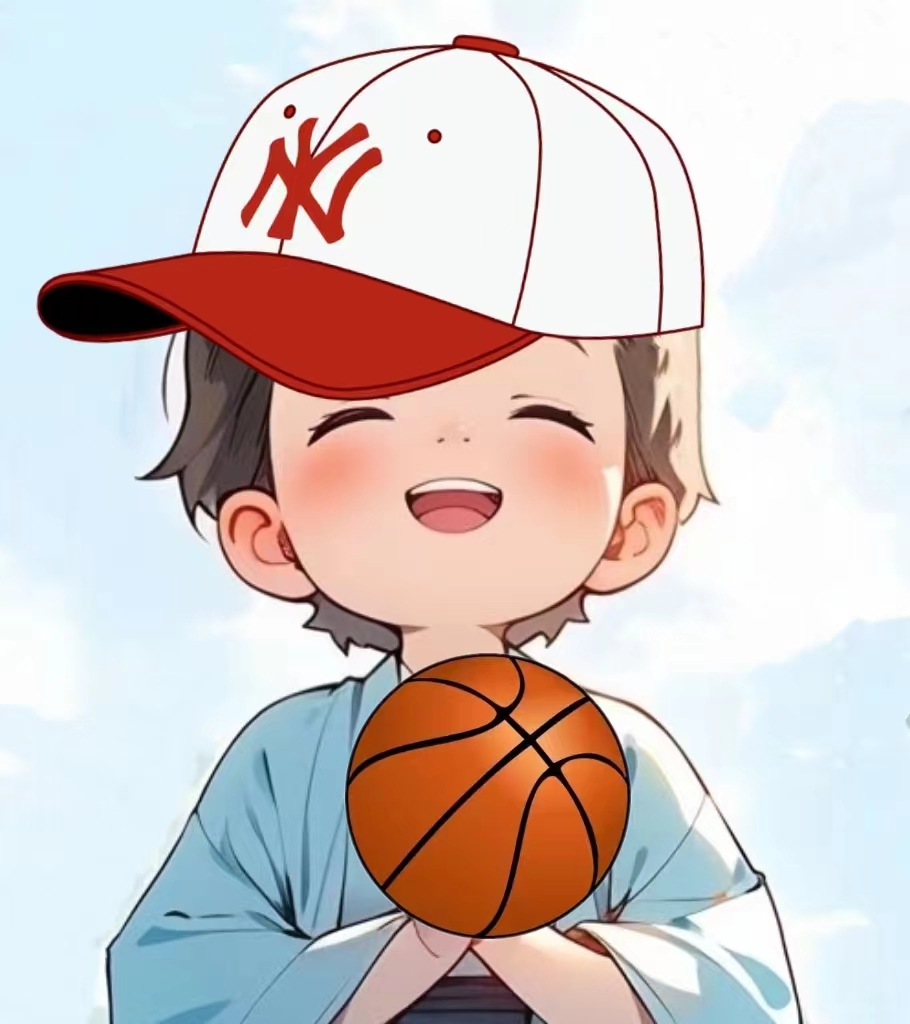
CNN+LSTM
该代码实现了一个由四个卷积层、两个LSTM层和一个ResNet模块组成的神经网络。首先使用四个卷积层提取图像特征,然后将残差块的输出通过LSTM层进行处理。将LSTM层的输出通过ResNet模块进行处理,最后再次使用LSTM层输出最终结果。
four layers of Conv2d + one layer of LSTM
conv2d_layer
函数实现了卷积层
1 | def conv2d_layer(inputs, filters, kernel_size, strides, padding): |
residual_block
函数实现了残差块
1 | def residual_block(inputs, filters, kernel_size, strides, padding): |
lstm_layer
函数实现了LSTM层
1 | def lstm_layer(inputs, hidden_size, num_layers): |
完整实现
1 | # 输入数据 |
ResNet + one layer of LSTM
完整实现
1 | # 将LSTM的输出转换为ResNet的输入 |
3D CNN
该代码段实现了一个由三个卷积层、一个3D ResNet模块和一个ResNet (2+1)D模块组成的神经网络。分别对应了Isolated Sign Language Recognition在github上的三种实现方法。
three layers of Conv3d
conv3d_layer
函数实现了3D卷积层
1 | def conv3d_layer(inputs, filters, kernel_size, strides, padding): |
residual_3d_block
函数实现了3D残差块
1 | def residual_3d_block(inputs, filters, kernel_size, strides, padding): |
residual_2plus1d_block
函数实现了(2+1)D残差块
1 | def residual_2plus1d_block(inputs, filters, kernel_size, strides, padding): |
3D ResNet
three_layers_3d_resnet
函数实现了3D ResNet
模块
1 | def three_layers_3d_resnet(inputs): |
ResNet (2+1)D
three_layers_2plus1d_resnet
函数实现了ResNet (2+1)D
模块
1 | def three_layers_2plus1d_resnet(inputs): |
three_layers_3d_resnet_2plus1d
函数将两个模块组合在一起。
1 | def three_layers_3d_resnet_2plus1d(inputs): |
定义输出层和损失函数及优化器
1 | # 输出层 |
具体工作
在github该项目检索发现ResNet(2+1)D神经网络识别率准确率均最高,所以对这个网络类型展开调研,具体实现函数即上面所写的three_layers_2plus1d_resnet
函数,内容如下:
主要内容
ResNet (2+1)D神经网络是一种用于视频分类的卷积神经网络,它是ResNet在时间维度上的扩展。
ResNet (2+1)D网络的基本思想是在2D卷积和1D卷积之间交替进行,以分别处理空间和时间维度上的特征。在这种网络结构中,每个卷积层都包括一个2D卷积和一个1D卷积,其中2D卷积用于处理空间维度上的特征,1D卷积用于处理时间维度上的特征。这种结构使得网络能够同时考虑空间和时间维度上的特征,从而更好地处理视频数据。
此外,ResNet (2+1)D网络也采用了ResNet的残差连接思想,在每个2D卷积层和1D卷积层之间添加了残差连接,使得网络能够更深,并且可以避免梯度消失问题。 ResNet (2+1)D网络在视频分类等任务上取得了很好的结果,尤其是在UCF101和HMDB51等数据集上的表现优异。该网络的训练和使用也非常简单,只需对视频进行帧采样和数据增强,然后将其输入到网络中进行训练即可。
在项目中的对应
ResNet(2+1)D神经网络依靠CSL_Isolated_Conv3D这个程序实现,类定义为r2plus1d_18
函数(调用对应文件Conv3D.py
的519行,实现对应文件Conv3D.py
的480行
在项目中这部分直接调用torchvision.models.video.r2plus1d_18
函数,看不到底层实现。(所以应该可以调用上面tenserflow写出来的实现函数)
- Title: tensorflow-model-summary
- Author: Charles
- Created at : 2023-03-21 13:36:47
- Updated at : 2023-07-27 16:45:54
- Link: https://charles2530.github.io/2023/03/21/tensorflow-model-summary/
- License: This work is licensed under CC BY-NC-SA 4.0.