Cpp_part2
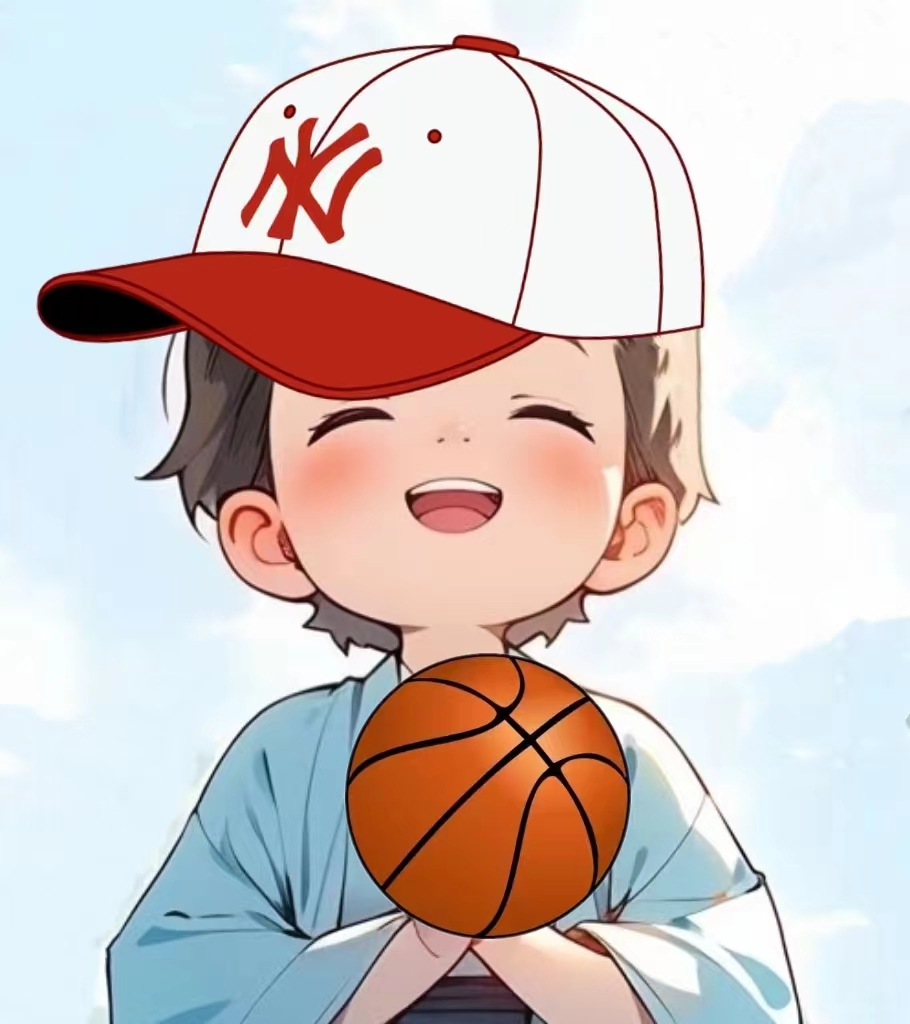
Cpp_part2
1.exception 异常处理
1 | void fun1(int){}; // 占位符,程序员必须要传 |
2.类和对象
C语言能面向对象吗?
一方面everything is object #C语言能面向对象
另一方面封装,继承,多态 #C语言不能面向对象
结构是相关属性的集合,与结构相关的操作均独立于结构之外
1 | struct Student { |
故C语言解决了封装,但封装的不彻底
1 | class Student { |
类是抽象的,对象是具体存在
类是唯一的,对象是无穷多的
类中函数不会占有对象空间
一个对象就是一段连续的内存
Main part
库程序员,终端程序员
面向对象站在库程序员的角度
1 |
|
- Title: Cpp_part2
- Author: Charles
- Created at : 2022-12-28 08:08:31
- Updated at : 2023-02-09 17:57:02
- Link: https://charles2530.github.io/2022/12/28/cpp-part2/
- License: This work is licensed under CC BY-NC-SA 4.0.
recommend_articles
recommend_articles
Comments